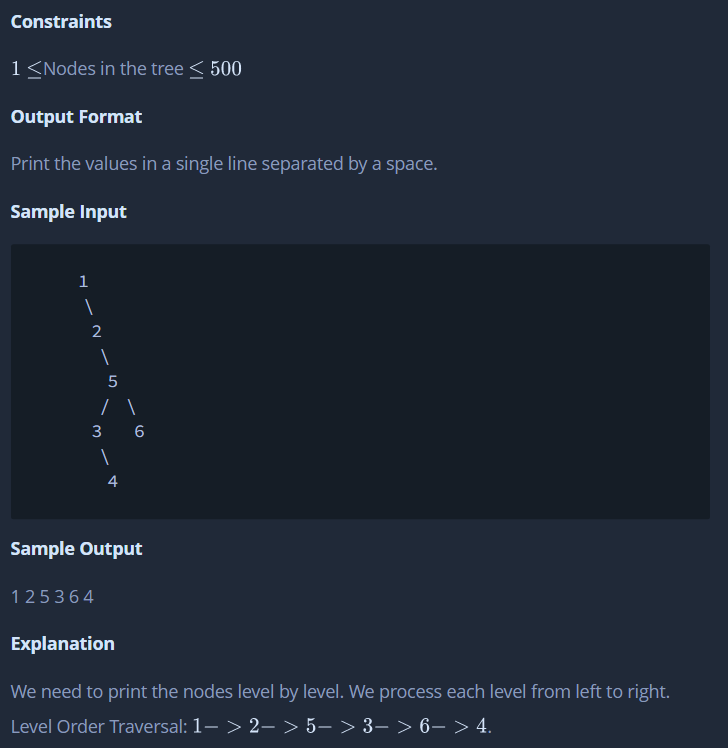
https://www.hackerrank.com/challenges/tree-level-order-traversal/problem Tree: Level Order Traversal | HackerRank Level order traversal of a binary tree. www.hackerrank.com Data Structures > Trees 트리가 주어지면 level order로 출력한다. 코드 struct node* queue[500]; int front = -1, rear = -1; void addq(struct node *ptr){ queue[++rear] = ptr; } struct node* deleteq(){ return queue[++front]; } void levelOrder(s..
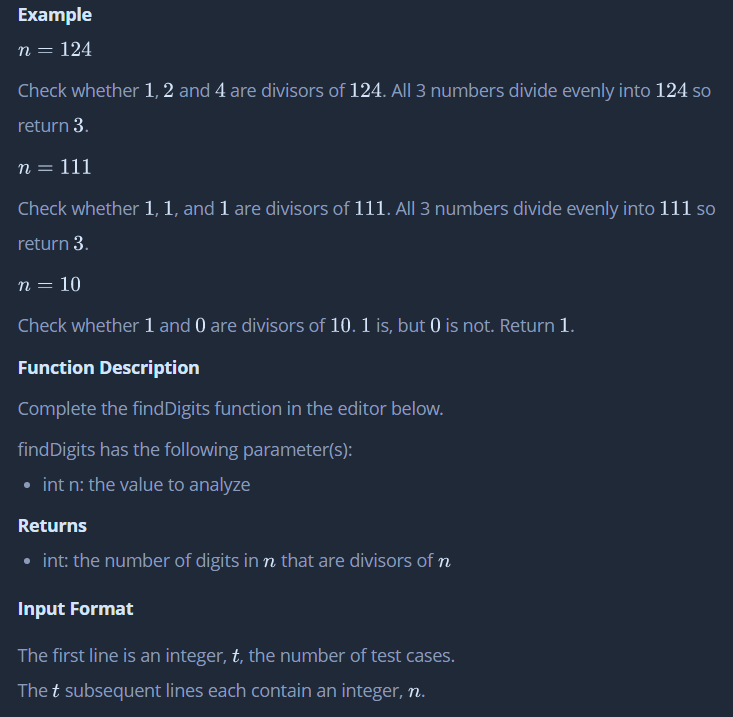
https://www.hackerrank.com/challenges/find-digits/problem Find Digits | HackerRank Calculate the number of digits in an integer that evenly divide it. www.hackerrank.com Algorithms > Implementation 정수 n이 주어지면 각 자릿수가 n의 약수인지 판별한다. 코드 int findDigits(int n) { int result = 0, now = n; while (now){ if (now % 10 == 0){ now /= 10; continue; } else if (n % (now % 10) == 0) result++; now /= 10; } return ..
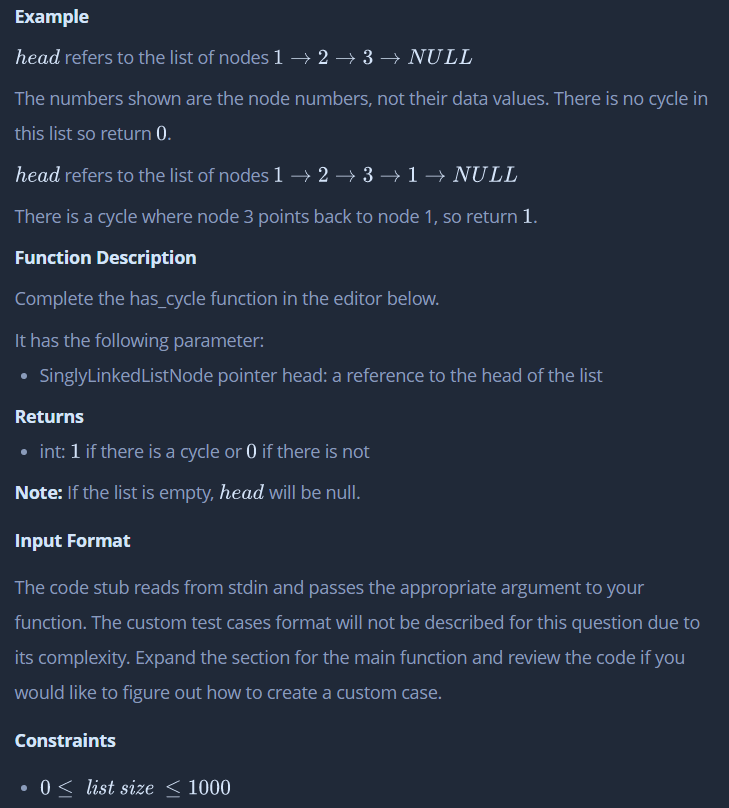
https://www.hackerrank.com/challenges/detect-whether-a-linked-list-contains-a-cycle/problem Cycle Detection | HackerRank Given a pointer to the head of a linked list, determine whether the linked list loops back onto itself www.hackerrank.com Data Structures > Linked Lists 연결 리스트의 head가 주어지면 해당 리스트가 순환 되는지 체크한다. 코드 bool has_cycle(SinglyLinkedListNode* head) { SinglyLinkedListNode* first = head, ..
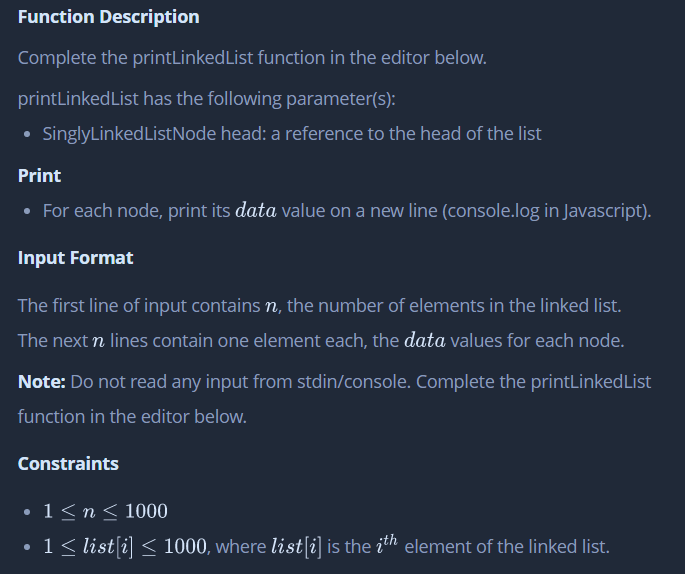
https://www.hackerrank.com/challenges/print-the-elements-of-a-linked-list/problem Print the Elements of a Linked List | HackerRank Get started with Linked Lists! www.hackerrank.com Data Structures > Linked Lists 연결 리스트가 주어지면 각 데이터를 출력한다. 코드 void printLinkedList(SinglyLinkedListNode* head) { if (!head) return; printf("%d\n", head->data); printLinkedList(head->next); } 설명 재귀를 이용했다. 처음에 head가 NULL인..